How to find If a File exists using PHP?
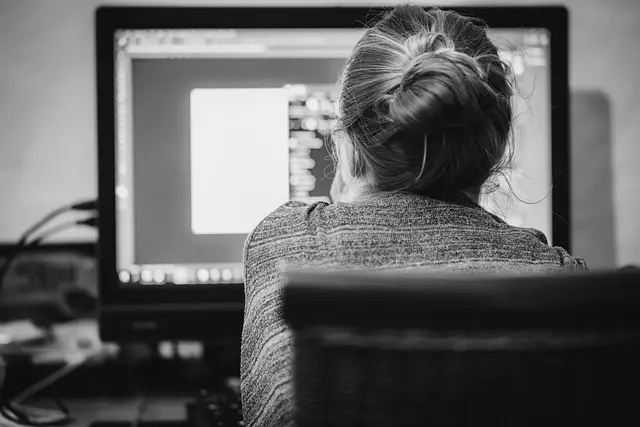
Determining if a file is available is a common task in PHP. There are 2 different methods available in PHP to check if file exists in a path.
Both these function can be used within a IF condition. Lets see how to use them.
1. PHP check if File exists
In PHP, check if a file exists in a folder path – using the function: file_exists()
, which returns true if file is present in the specified folder & false otherwise.
Syntax: file_exists (‘filepath’)
This fucntion takes the input as file along with complete path to this function.
Here is the detailed php code example:
// Define file path
$pathtofile = '/folder1/subfolder1/filename.ext';
// Clear cache to remove result from previous run
clearstatcache();
// Check if file is present & Returns True or False
echo file_exists($pathtofile);
// How to use this file_exists in If condition
if (file_exists($pathtofile)) {
echo "File found in directory path";
} else {
echo "File not found in the path";
}
The return code can be printed or assigned to a Boolean variable & used inside your php code.
2. PHP check if File Exists using Is_File?
Another way to check if a file exists in a folder is by using the is_file()
function, which returns true if the specified file path is a regular file, and false otherwise.
// Define file path
$pathtofile = '/folder1/subfolder1/filename.ext';
// Clear cache to remove result from previous run
clearstatcache();
// Check if file is present & Returns True or False
echo is_file($pathtofile);
// Using is_file function within If condition
if (is_file($pathtofile)) {
echo "File found in directory path";
} else {
echo "File not found in the path";
}
In both these functions the parameter and the returning value are same.
There is no difference in the implementation of these 2 options. But, before using this function also, read about the notes section towards the end of this article.
3. Additional Code – How to Check if Folder exists in PHP?
Well, we have seen the file to check if file exists using PHP. What if we need to check whether the folder itself is missing or not?
Use this code snippet that can be handy.
// Define folder path
$pathtofolder = '/folder1/subfolder1/';
// Clear cache to remove result from previous run
clearstatcache();
// Check if file is present & Returns True or False
echo is_dir($pathtofolder);
// How to use this is_dir function in If condition
if (is_dir($pathtofolder)) {
echo "Folder found in path";
} else {
echo "Folder not found in the path";
}
Important Note: There is chance that even if the file is moved to a different place, the function still returns a previous return code. It is because the function uses cache.
It is always a good practice to use ‘clearstatcache()’ prior to using the file management functions. That way, we will always get the latest result.
External Reference:
Related Topics that might interest you.