In our digitally driven world, where data processing and management are integral to many industries, the ability to work with Python has become an invaluable skill. This language, particularly in relation to file and directory manipulation, grapples with the rapid growth of data and its intricate handling. With its intuitive syntax, robust set of built-in functions, and vast standard library, Python simplifies tasks such as reading, writing, and manipulating text files and directories. This discussion delves into the fundamental aspects of Python, including data types, conditional statements, loops, and functions, before exploring the versatile os module for interacting with the operating system. Additionally, this discourse emphasises the importance of understanding and handling common errors in Python, a crucial aspect of efficient and effective programming.
Python Basics
Mastering File Management in Python: Key Concepts Demystified
Python, celebrated for its versatility and efficiency, is a robust programming language that appeals to beginners and experts alike. This article will guide you through the crucial Python concepts necessary for competent file operations, all in a language that’s easy to understand and immediately applicable. With Python’s powerful and intuitive syntax, automating file handling processes will be second nature in no time.
To start, let’s explore the two prevalent categories of file types in Python – text files and binary files. Text files contain data in a human-readable format like .txt or .csv, while binary files contain computer-readable data like .jpg or .exe. Understanding these file types allows Python enthusiasts to plan the appropriate approach to reading or writing data.
Now, on to the core file operations – open, read, write, and close. When working with files in Python, the method 'open()'
is indispensable. It opens a file and returns a file object, which is then used for further operations. Here’s a nifty trick, if the file you are trying to open doesn’t exist, Python creates one automatically!
The 'read()'
function enables you to extract data from a file. For instance, using the 'read()'
function without a parameter returns the entire content of the file, while an integer parameter reads a specified number of characters.
On the flip side, the 'write()'
function allows you to add data to a file. What’s interesting is the usefulness of two types of write functions: 'write()'
and 'writelines()'
. The 'write()'
function expects a string as a parameter, while 'writelines()'
can take a list of strings.
Lastly, it’s essential not to neglect the 'close()'
function. It’s recommended to close files after they’ve served their purpose, as it might cause unexpected behavior or consume unnecessarily high amounts of system resources.
Let’s spice things up with Python’s input-output modes. The 'r'
mode reads a file, the 'w'
opens a file for writing, the 'a'
appends data to an existing file, and the 'x'
creates a new file but throws an error if the file exists. Handy, right?
Moreover, Python goes an extra mile with 'seek()'
and 'tell()'
functions. The 'tell()'
method returns the current position of the file read/write pointer within the file while 'seek()'
method moves the file read/write pointer to a given specific position.
Sailing further, we encounter file exceptions. Python might throw an 'IOError'
if the file cannot be opened, or a 'FileNotFoundError'
if the file does not exist. Cautious coding can help catch these exceptions and avoids your program from prematurely crashing.
In the world of Python programming, wrapping file operations within 'with'
statements ensures that files are properly closed after operations are completed, even if an exception is raised. This practice not only enhances your file handling process but is also a testament to your proficiency in Python.
Finally, let’s glance at file handling libraries. Modules such as 'os'
, 'shutil'
, and 'glob'
can automate mundane tasks like changing file paths, copying or moving files, and finding all paths matching a certain pattern.
In conclusion, Python’s file handling capability is indeed a game changer. Whether it’s working with text or binary files, reading or writing data, catching file exceptions or using file handling libraries, Python’s comprehensive toolbox empowers tech enthusiasts to transform file management into an efficient, automated, and precise process. So, let’s embrace these concepts, apply them, and elevate the Python programming adventure!
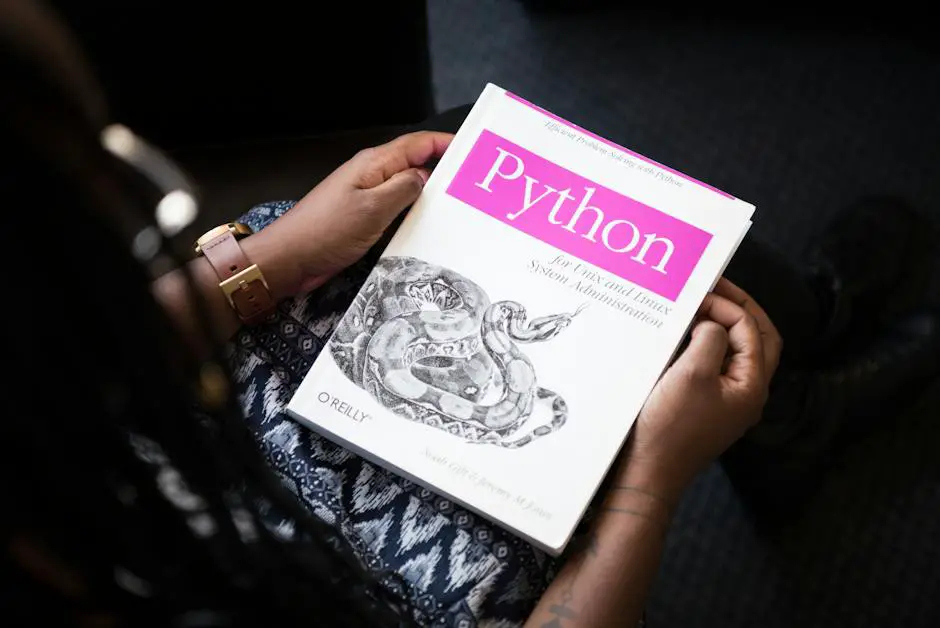
Working with Directories in Python
Python has an extensive set of built-in libraries; among these libraries is the ‘os’ module, an incredibly versatile tool well-suited for handling files, directories and other operating system functionalities. Let’s delve into the practical application of the ‘os’ module to get a better grip on directory manipulation.
The ‘os’ module provides a plethora of built-in methods for creating, removing, and changing directories. Some of the main functions include os.mkdir()
, os.rmdir()
, and os.rename()
for creating, deleting, and renaming directories, respectively. To use these functions, you must first import the ‘os’ module using the command ‘import os.’
The os.mkdir()
function allows Python programmers to generate new directories. This function requires the name of the directory to be created as a parameter. For instance, os.mkdir("testdir")
creates a new document called “testdir”.
Deleting directories is equally straightforward. The os.rmdir()
function will erase a specified directory permanently. An important caveat to remember though, this only works for empty directories. This can be executed with os.rmdir("testdir")
to remove the previously established “testdir”.
Renaming directories is yet another useful function of the ‘os’ module. With os.rename()
, you can change the name of your directories. To illustrate, os.rename("initial_dir","new_dir")
will rename a directory named “initial_dir” to “new_dir”.
Prudently managing directories means beyond creating, deleting, and renaming them; ideally, you should also know how to navigate within these directories. The os module gives two robust functions for this: os.getcwd()
and os.chdir()
.
With os.getcwd()
function, you can access the current working directory path, allowing you to grasp your current location in the file system. An instance of this could be print(os.getcwd())
, which prints the path of the current working directory.
To change the current working directory to another directory, the function os.chdir()
is utilised. For instance, os.chdir("/path/to/your/directory")
will change the current working directory to “/path/to/your/directory”.
The ‘os’ module also allows us to traverse directories, similar to using ‘ls’ command in Unix. os.listdir()
returns a list containing the names of all the entries in the directory given by the path. The list is in arbitrary order and does not include the special entries ‘.’ and ‘..’ even if they are present in the directory.
In conclusion, Python’s os module opens up a host of avenues for proficient navigation and manipulation of directories. These powerful tools can be quite instrumental in developing file management systems, automating routine tasks, and progressing your Pythonic journey. The best way to master these tools is hands-on experimentation. So, roll up your sleeves and start coding! Remember, a well-structured directory is the backbone of any successful programming project!
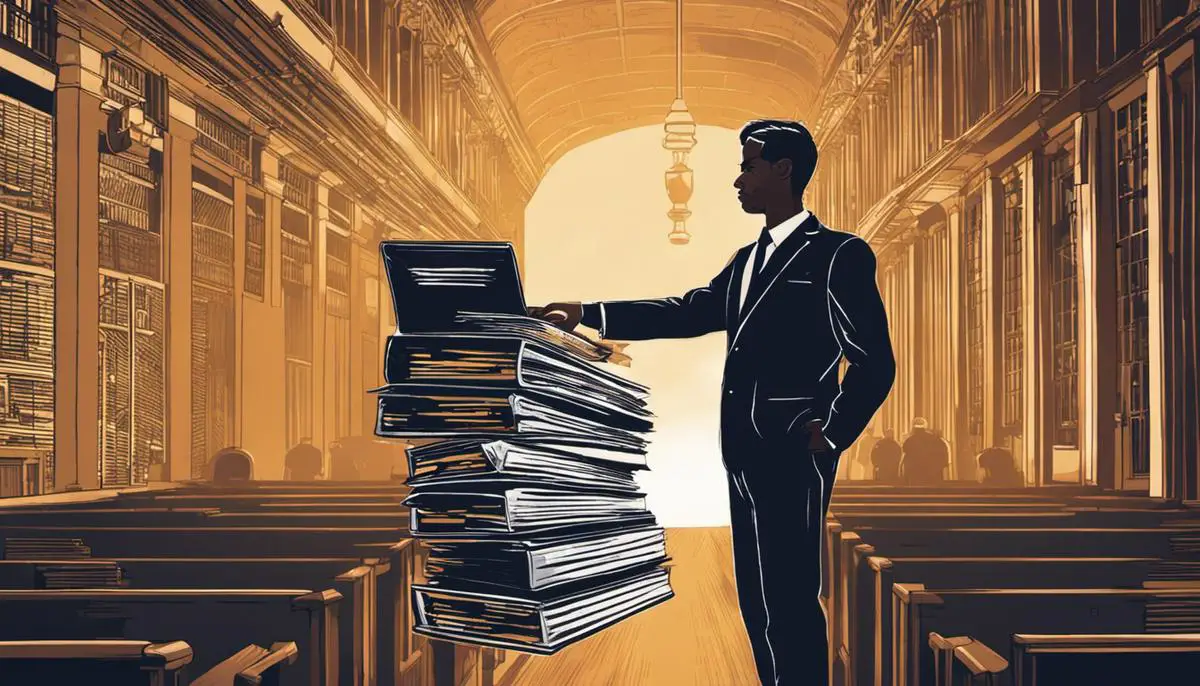
Error Handling in Python
Moving forward in understanding Python's mechanism for managing files and directories, let's delve into how to handle exceptions and errors effectively.
Breaking down error when manipulating files in Python, the two most common types we encounter are: SyntaxError (when Python parser encounters syntax not within the grammar rules) and Exception (when the syntax is correct but the operation is incorrect). Each of these error types have sub-types which occur in different scenarios. Particularly for file handling, some common exceptions are: FileNotFoundError, PermissionError, NotADirectoryError, and IsADirectoryError.
A bullet-proof method to handle exceptions is to use 'try' and 'except' statements. Think of a 'try' block as an operation you want to execute, and an 'except' block as a backup plan if that operation fails. This allows the code to continue execution even if an error or exception occurs. Below is a general form:
try: # Operation to be performed
except (ExceptionName1, ExceptionName2, ...): # What to do in case of this exception
For instance, when opening a file that doesn't exist, a FileNotFoundError would ordinarily halt the program. By using 'try' and 'except', we can give the program instructions on what to do when this happens, like this:
try: file = open('non_existent_file.txt', 'r') except FileNotFoundError: print('File does not exist. Please check the file path and try again.')
PermissionError occurs normally when we try to write or delete a file we do not have access rights to. Similar to FileNotFoundError, we can better manage the impacts of the error with 'try' and 'except':
try: file = open('important_file.txt', 'w') except PermissionError: print('You do not have sufficient permissions to write to this file.')
When we're interacting with directories, it's typical to encounter NotADirectoryError and IsADirectoryError. The former happens when we use a file for an operation meant for a directory, while the latter occurs when we treat directories as files.
Let's see how to handle a NotADirectoryError:
try: os.chdir('some_file.txt') # A change directory operation on a file except NotADirectoryError: print('Specified path does not lead to a directory.')
And similarly, an IsADirectoryError:
try: file = open('some_directory', 'r') # Trying to open a directory as if it were a file except IsADirectoryError: print('The specified path is a directory, not a file.')
Don't forget to incorporate these error handling practices in Python programming for more robust and failure-resistant applications. On a final note, remember that creating good directory structures in your projects is a key step towards minimizing such errors. These structures would provide a roadmap for Python to follow as it navigates files and directories, making error management much easier. Happy coding!
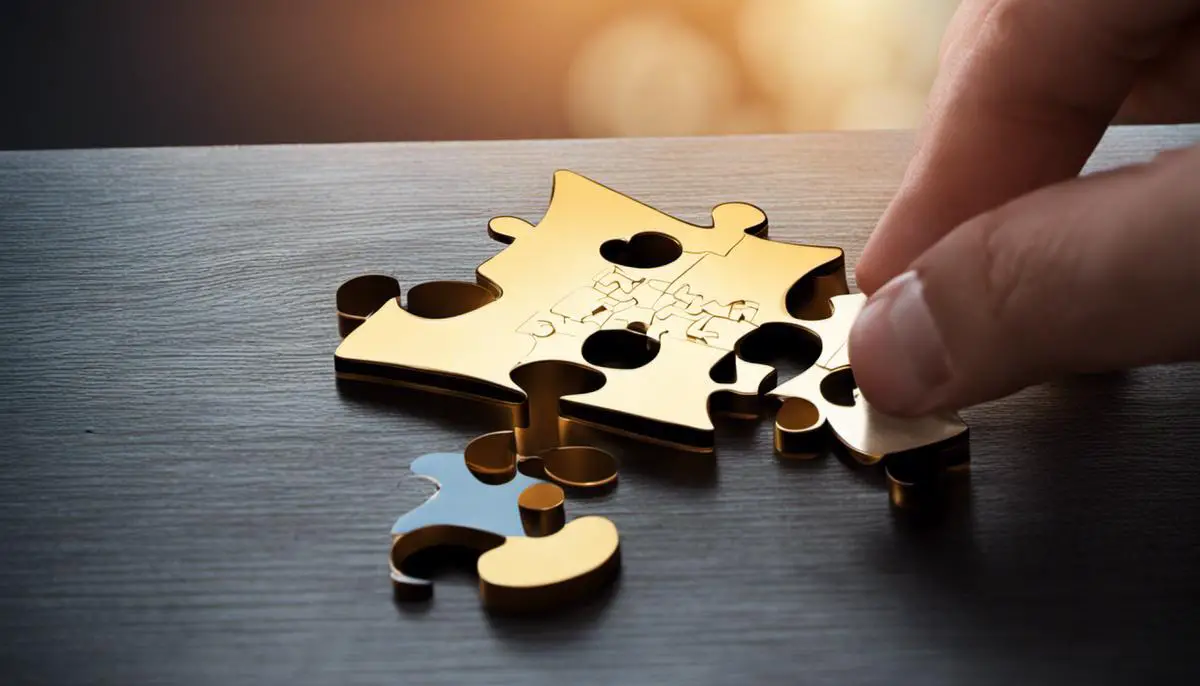
Mastering the elements of Python discussed here not only equips individuals with the ability to interact with files and directories but also cultivates a strong foundation for tackling more complex programming challenges. Python’s os module and built-in functions abstract many of the complexities of dealing with the filesystem, rendering it easier to perform routine tasks. By recognizing common errors and implementing proper error handling, one can write robust programs that can withstand real-world testing scenarios. As we traverse further into the information age, the importance of cultivating these skills cannot be overstated. Armed with Python knowledge, anyone is well-equipped to adapt and thrive in a constantly evolving digital landscape.