What is AJAX?
AJAX stands for asynchronous javascript and xml. It is a technique where we can request data from the server without reloading the entire page, it is not a programming language, it is a combination of different technologies listed below
Language used in ajax
- Html
- Css
- Javascript
- Php
- Xml
- Json
In ajax the word asynchronous means exchanging data to/from the server in the background without having to refresh the page.
The main components of the ajax are as followed:
- A browsers built in XMLHttpRequest oject (to request data from the webserver)
- Javascipt and html dom (to display the data).
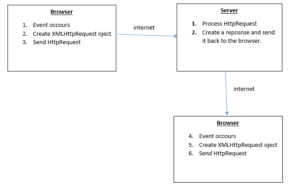
Example:
<script>
function addUser() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function () {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("demo").innerHTML =
this.responseText;
}
};
xhttp.open("GET", "update.php", true);
xhttp.send();
}
</script>
In this code snippet above there is a function called addUser() which is used to add a given user to the database
A variable xhttp is defined which holds the object XMLHttpRequest
It checks for the change in state of the page, using the property onreadystatechange and it defines a function to be called when the ready state property is changed
On readystatechnge function is called every time the readystate changes
Initializing this state change to a function which checks for the status value
Here 4 means request is finished and response is ready and 200 means it is OK
The readystate values are:
- 0: request not initialized
- 1: server connection established
- 2: request received
- 3: processing
- 4: request is finished and response
The status values are:
- 200: OK
- 403: Forbidden
- 404: page not found
xhttp.send() : sends the request to the server. Used for Get requests.
xhttp.open() : specifies the page to be opened using method “GET” or “POST” or is it synchronous or asynchronous(true or false).
XMLHttpRequest object has several different methods:
- new XMLHttpRequest(): creates a new XMLHtttpRequest object.
- abort(): cancels the current request.
- getAllResponseHeaders(): Returns header information.
- getResponseHeader(): returns specific header information.
- setRequestHeader(): adds a label value pair to the header to be sent.