strftime
For quick syntax & options help with this function, type >>help (“time.strftime”) . This will list all the basic help that you are in need. If you are looking for a much more advanced option with this function, then refer this table.
About this function: This function is used to format the date & time returned from gmtime() or localtime() functions.
where ‘format’ is a combination of the directives listed below and it can be a combination of more than one directive passed as a string parameter. Lets see an example & then the list of all possible directives.
time.strftime example:
>>from time import gmtime, strftime >>> strftime("%a, %d %b %Y %H:%M:%S") 'Wed, 13 Dec 2017 22:59:26' >>> strftime("%a, %d %b %Y %H:%M:%S",gmtime()) 'Wed, 13 Dec 2017 17:29:36' >>>
Note: Second Parameter is optional. If left blank, time from localtime() will be considered.
Directives & their meaning:
Derivative | Description | Example |
---|---|---|
%a | Weekday as locale’s abbreviated name. | Sun |
%A | Weekday as locale’s full name. | Sunday |
%w | Weekday as a decimal number, where 0 is Sunday and 6 is Saturday. | 1 |
%d | Day of the month as a zero-padded decimal number. | 31 |
%-d | Day of the month as a decimal number. (Platform specific) | 31 |
%b | Month as locale’s abbreviated name. | Jan |
%B | Month as locale’s full name. | January |
%m | Month as a zero-padded decimal number. | 1 |
%-m | Month as a decimal number. (Platform specific) | 1 |
%y | Year without century as a zero-padded decimal number. | 17 |
%Y | Year with century as a decimal number. | 2017 |
%H | Hour (24-hour clock) as a zero-padded decimal number. | 10 |
%-H | Hour (24-hour clock) as a decimal number. (Platform specific) | 10 |
%I | Hour (12-hour clock) as a zero-padded decimal number. | 10 |
%-I | Hour (12-hour clock) as a decimal number. (Platform specific) | 10 |
%p | Locale’s equivalent of either AM or PM. | AM |
%M | Minute as a zero-padded decimal number. | 5 |
%-M | Minute as a decimal number. (Platform specific) | 5 |
%S | Second as a zero-padded decimal number. | 5 |
%-S | Second as a decimal number. (Platform specific) | 5 |
%f | Microsecond as a decimal number, zero-padded on the left. | 0 |
%z | UTC offset in the form +HHMM or -HHMM (empty string if the the object is naive). | |
%Z | Time zone name (empty string if the object is naive). | CDT |
%j | Day of the year as a zero-padded decimal number. | 365 |
%-j | Day of the year as a decimal number. (Platform specific) | 365 |
%U | Week number of the year (Sunday as the first day of the week) as a zero padded decimal number. All days in a new year preceding the first Sunday are considered to be in week 0. | 25 |
%W | Week number of the year (Monday as the first day of the week) as a decimal number. All days in a new year preceding the first Monday are considered to be in week 0. | 25 |
%c | Locale’s appropriate date and time representation. | Sun Jan 31 10:05:05 2017 |
%x | Locale’s appropriate date representation. | 1/31/2017 |
%X | Locale’s appropriate time representation. | 10:05:05 |
%% | A literal ‘%’ character. | % |
Source Reference: This table is referred from the page strftime.org . This page only has one page with description about this function alone. The author has created this page since a quick reference.
From Python IDE
This is also a quick ref image for the command syntax & its parameters.
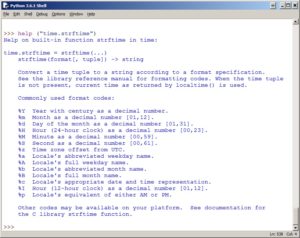
C or Python strftime
Python function strftime() call the same function from the c Library. It is just that it has a slightly different syntax. Other than that, the directives used as parameter are all the same.
Explanation for the Parameters:
- *str – Pointer to the output string
- maxsize – Size of the above output string
- format – same as Python strftime directive
- *timeptr – Pointer to input calendar time.
For the struct tm, the structure can be seen in time.h header file.
Sample code with strftime C
#include <stdio.h> #include <time.h> void main () { time_t systime; struct tm *calendartime; char formattedtime[60]; time( &systime ); calendartime = localtime( &systime ); strftime(formattedtime,60,"%a, %d %b %Y %H:%M:%S", calendartime); printf("Formatted date & time : |%s|\n", formattedtime ); }
Reference Links:
Some of the codes are copied, tested & modified a bit from the above links & posted here as a collection for a quick reference.