VBA Code to Sort Outlook Emails by Date
In Outlook app, when you are viewing the mails in any folder, You have option to Sort them based on Received date/time, Sender, Subject etc.,
But in Outlook VBA code, when we retrieve emails, we may not be sure on which order the emails are being retrieved. To ensure that it is sorted based on certain criteria, we have to explicitly mention the criteria & order (ascending or descending).
Let see how to do this in a VBA code.
Outlook Macro to Sort Emails by Date
Lets assume that you would like to read the latest emails in the Inbox first. To do this, the emails should be sort by date in descending order.
The following code just does that. It sorts all the emails in a specific folder by date (received time).
Function Sort_Inbox_By_Date()
'Define
Dim objInboxFolder As Folder
Dim objMailItem As MailItem
Dim objItems As Items
Dim objItem As Object
Dim itmCount As Double
'Init
Set objInboxFolder = Application.GetNamespace("MAPI").PickFolder
'Sort
Set objItems = objInboxFolder.Items
objItems.Sort "[ReceivedTime]", True
'Loop thru each Item after Sort
itmCount = 0
For Each objItem In objItems
If TypeName(objItem) = "MailItem" Then
itmCount = itmCount + 1
Set objMailItem = objItem
Debug.Print itmCount & ": " & objMailItem.ReceivedTime & " - " & " - " & objMailItem.SenderEmailAddress
End If
Next
'Done
MsgBox "Sort completed"
End Function
Now, lets see how this works. Before sorting the results would look like this and after soring the same code reads the first mail in different order like in this image.
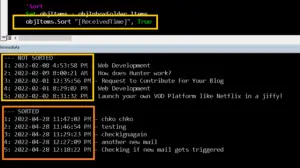
There are two parameters to this .Sort function. It is the email field that we would like to perform sort on.
In this case we are sorting based on received time. And the next one is the descending field. It is an optional field and is set to False by default. So, if you dont mention anything the emails will be sorted by older emails first.
Here are some additional reference.
- Email object parameters & explanation from Official website.